HTML/CSS: Introduction
HTML
HTML stands for HyperText Markup Language. HTML is used to create structure and separation of content on a web page.
HTML Tags
The basic building block of HTML is a tag, or element. A tag is written with two angle brackets <tag>. Most HTML tags come in pairs, with an opening version <tag> and a closing version </tag>. The closing version marks the end of the content of the tag. So, a typical HTML tag may look as follows:<tag>Content</tag>
Singleton Tags
There are a few HTML tags that do not have any content, and thus no closing partner. These are called singleton tags. Singleton tags are written with a / inside the tag to signal the computer not to expect a closing tag.<singletontag />
Attributes
Often, we need to provide extra information for a tag to be processed a certain way by the computer. This information comes in the form of attributes. An attribute is a name/value pair. HTML attributes separate the name and value with an = sign and enclose the value in single quotes. If there are multiple atttributes, simply place a space between the end of one and the beginning of another.<tag att1='value1'>Content</tag> <singleton att1='value1' att2='value2' />
Whitespace
HTML ignores all whitespace (spaces, tabs, new lines) beyond a single space. The only way to create a line break in HTML is to use a <br /> tag. Note that the br tag is a singleton and has no content.Headings
Not to be confused with the document head, the heading tags are numbered 1-6 based on size, where the h1 heading is the biggest and h6 is the smallest. The heading tags have pre-written styles that bold and size their content. Additionally, they have built in line breaks both before and after.<h1>Level 1 Heading</h1> <h2>Level 2 Heading</h2> <h3>Level 3 Heading</h3> <h4>Level 4 Heading</h4> <h5>Level 5 Heading</h5> <h6>Level 6 Heading</h6>
Level 1 Heading
Level 2 Heading
Level 3 Heading
Level 4 Heading
Level 5 Heading
Level 6 Heading
Structure of an HTML Page
<!DOCTYPE html> <html> <head> <title>Page Title</title> </head> <body> <h1>Welcome!</h1> This is the first line.<br /> This is the second line. </body> </html>Line Number Descriptions:
- The DOCTYPE command lets the computer know what type of information to expect. In this case, it is expecting HTML
- The html tag begins the html information. It is paired with the end of the html information on line 11. ALL content on the page should be within these two tags.
- The head section of the document is used for storing information about the document. NO VISIBLE ELEMENTS should be in the head. This tag pairs with its ending tag found on line 5. The head section is one of two direct descendents of the html tag.
- The contents of the title tag are displayed on the tab of the web page, but not on the web page itself. The content of the title tag is also used by search engines to help identify page content.
- The end of the head section.
- The body of the document is where ALL of the VISIBLE CONTENT should be placed. This tag pairs with its ending tag found on line 10. The body section is one of the two direct descendents of the html tag.
- The h1 tag is the largest of the heading tags. The content inside this tag will be automatically sized, bolded and will have built in line breaks before and after it.
- A line of output, that includes a line break tag to force output to the next line.
- A second line of output.
- The end of the body tag.
- The ending html tag signifying the end of the document.
HTML Comments
Comments are used by programmers to leave notes, instructions, dividers, etc in their code. These comments are not intended for the view to see, but only the programmers of the page. An HTML comment is denoted with <!-- -->. This comment can span one or multiple lines.CSS
CSS stands for Cascading Style Sheets. CSS is used to style the HTML of a web page giving it color and design.
CSS Syntax
Styles are then set using CSS properites. CSS properties are specified with a name and value. The name and value pair are separated by a colon(:). If multiple properties are being assigned to the same rule, they are separated by a semicolon(;).color:blue; background-color:green;
Internal CSS
Internal CSS is written in style tags inside the head of the document. CSS is applied to the page using rules. A rule defines the element(s) that a set of properties are assigned to. The most basic type of rule is a tag name. Tag rules use the HTML element name (no angle brackets). After the rule, a set of curly braces is used to define the CSS properties to be assigned.body{ color:white; background-color:blue; } h1{ color:pink; }In the above example, the whole document (body) will use white text on a blue background. All h1 elements will be pink.
CSS Colors
Colors can be set in css using reserved color words or hex codes. When using hex codes, be sure to include the '#' at the start.CSS Comments
Comments are used by programmers to leave notes, instructions, dividers, etc in their code. These comments are not intended for the view to see, but only the programmers of the page. A CSS comment is denoted with /* */. This comment can span one or multiple lines.Putting it all together
<!DOCTYPE html> <html> <head> <title>Page Title</title> <style> body{ color:white; background-color:blue; } h1{ color:pink; } </style> </head> <body> <h1>Welcome!</h1> This is the first line.<br /> This is the second line. </body> </html>
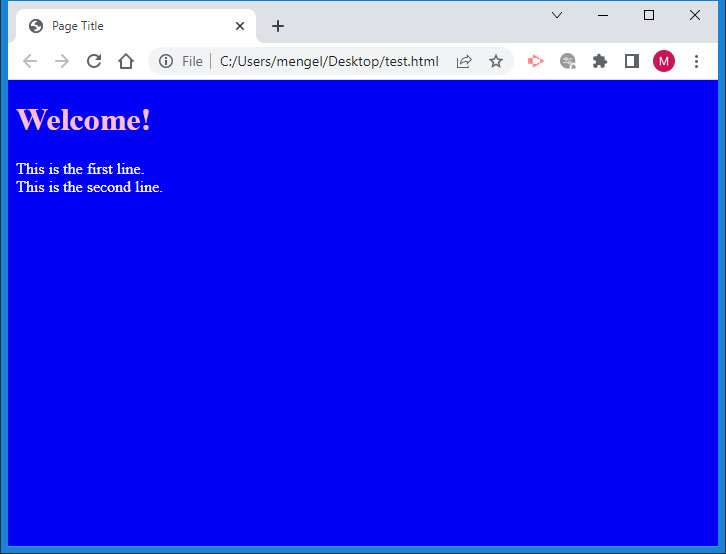